For a few weeks now I had the problem that the microphone level in windows kept going down. Wen I set it to 100% and look a few minutes later its at 96 % or lower.
I did unset the exclusive-mode uder advances with no avail.
So I did what any sane person does: I wrote a software which detecs the change and resets it to my desired level!
Sourcecode: https://gitlab.efana.de/sheepchen/MicAdjuster
Programm: https://nextcloud.sheepchen.net/index.php/s/AZyiam3rE5pii6D (compiled win_x64)
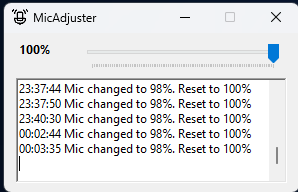
The Program reads in the current level at start. After this it will keep the level you tell him.
Every second it checks the windows setting against your desired value and changes it if it differs.
Minimize minimizes it into the NotifyTray. If you wan’t to get rid of it, just quit it. The is no installer, config, registry or something like that.
And in my case: I found out that it was the game Helldivers 2 !